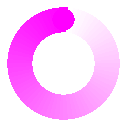
Python Tuples - Tuple and NamedTuple Type Tutorial with Examples - APPFICIAL
A tuple is like an immutable list, which means that it’s elements cannot be changed. Tuples have similar sequence type functions to lists, such as len. Tuples are created by creating a comma-separated list of values, surrounded by parenthesis. Ex:
disneyland_coordinates = (33.81, 117.92)
friends = (‘Ray’, ‘Alex’, ‘Mirna’)
total_friends = len(friends) # returns 3
Lists are more common than using a tuple. Only use tuples if you want to make sure the values won’t change. For example, the Disneyland coordinates should never change. But in the friends tuple, I may decide not to be friends with Ray anymore and I cannot change or remove him because I put him in a tuple.
Also, element position is important in tuples. In the Disneyland coordinates, we know that the first element is latitude, and second is longitude.
A named tuple creates a new data type with some named attributes. It does not create a new object, just a new simple data type. For example, a Person named tuple with fields like Person.name and Person.age would better represent a Person object than using a list. In the Person named tuple, name and age are the named attributes. Named tuples are also immutable, like regular tuples. To create a named tuple, add
from collections import namedtuple
to the top of your Python program. Here is an example:
Person = namedtuple(‘Person’,[‘name’,’age’,’weight’]) # create a named tuple called Person
bob = Person(‘Bob’, 45, 270.5) # use named tupble to describe a person
mary = Person(‘mary’, 36, 620.9) # use named tupble to describe a person
print(bob)
print(mary)
Subscribe to Appficial for more programming videos coming soon. Also, don't forget to click LIKE and comment on the video if it helped you out!
コメント