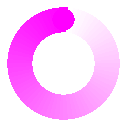
Effective Exception Handling in Spring Boot with @ControllerAdvice
Learn how to leverage Spring Boot's `-ControllerAdvice` annotation to catch exceptions from service classes effectively and enhance your application's error handling.
---
This video is based on the question stackoverflow.com/q/74666291/ asked by the user 'Leobejj' ( stackoverflow.com/u/20519796/ ) and on the answer stackoverflow.com/a/74666534/ provided by the user 'Iván Larios' ( stackoverflow.com/u/19839660/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to use -ControllerAdvice for catching exceptions from Service classes?
Also, Content (except music) licensed under CC BY-SA meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Effective Exception Handling in Spring Boot with -ControllerAdvice
When developing applications in Spring Boot, one common challenge developers face is managing exceptions that occur within service classes. You might find yourself in a situation where your service methods throw various types of exceptions, and you want to handle these errors gracefully. But how can you catch these exceptions effectively? The answer lies in using the -ControllerAdvice annotation.
Understanding the Problem
Imagine you have a service class with methods that throw exceptions, such as:
[[See Video to Reveal this Text or Code Snippet]]
In this scenario, when a request is made to retrieve an item by its ID, a situation may arise where the item does not exist in the database. If this exception is not handled properly, it can lead to unhelpful error messages being returned to users. This is where -ControllerAdvice can help you manage exceptions across your application's layers effectively.
What is -ControllerAdvice?
The -ControllerAdvice annotation in Spring allows you to define a global exception handler for your controllers. When any exception is thrown during the handling of a request, the controller marked with -ControllerAdvice intercepts it and allows you to define a response that should be returned to the client.
Implementing a Global Exception Handler
Step 1: Create a Global Exception Handler Class
To start using -ControllerAdvice, you'll need to create a dedicated exception handler class. Here’s a simple implementation:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Customize the Response
In the handleException method, you can customize the response that will be sent back to the client using a response entity. Here, we are using a hypothetical ApiError class that can hold useful information about the error:
HTTP Status: Indicate what type of error occurred (ex: NOT FOUND).
Error Message: Provide a clear message that describes the error.
Step 3: Using Custom Exceptions
You might also want to define custom exceptions that can make your error handling more robust. For example, creating a MyCustomException class for specialized errors:
[[See Video to Reveal this Text or Code Snippet]]
In your service layer, you can throw this exception when a specific error condition arises. Then, you can handle it in your global exception handler just like any other exception.
Benefits of Using -ControllerAdvice
Centralized Exception Handling: It allows you to manage exceptions in a single place, making the code cleaner and easier to maintain.
Consistent Error Responses: Your application will return a uniform error format, which improves the consumer experience.
Separation of Concerns: It separates error handling logic from business logic, allowing each layer of your application to focus on its primary responsibilities.
Conclusion
Using -ControllerAdvice for handling exceptions in Spring Boot is a powerful technique that enables you to manage errors from service classes effectively. By implementing a global exception handler, you can customize responses to ensure that users receive helpful and clear error messages, leading to a more robust application.
Remember to specify which exceptions you want to intercept and define the response accordingly. With this approach, you can enhance your application's error-handling strategy significantly.
Take charge of your exception handling today and build a better user experience!
コメント