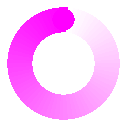
How to Exclude Imported Configurations from a Spring Boot Application in a @ SpringWebMvc Test
Learn how to easily exclude imported configurations from a Spring Boot application when writing @ WebMvc tests, ensuring a cleaner testing environment.
---
This video is based on the question stackoverflow.com/q/77049721/ asked by the user 'amsalk' ( stackoverflow.com/u/2496648/ ) and on the answer stackoverflow.com/a/77054980/ provided by the user 'Ken Chan' ( stackoverflow.com/u/339637/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Exclude imported configurations from Spring Boot Application in a @ SpringWebMvc test
Also, Content (except music) licensed under CC BY-SA meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Excluding Imported Configurations in Spring Boot @ WebMvcTest
When working with a Spring Boot application that consists of multiple modules, it’s common to import configurations from these modules into your primary application class. However, this can present challenges when you want to write a @ WebMvcTest for a @ RestController. If the configurations aren't handled correctly during tests, they might interfere with the application context, leading to unexpected behaviors. In this guide, we will discuss how to effectively exclude imported configurations during unit tests to ensure a clean testing process.
The Problem
Suppose you have a Spring Boot application like this:
[[See Video to Reveal this Text or Code Snippet]]
When you try to write a test for a @ RestController using @ WebMvcTest, you want to ensure that the configurations from FooConfiguration and BarConfiguration are not loaded. The goal is straightforward, but there are some caveats. You might have attempted to use:
@ WebMvcTest# excludeAutoConfiguration to exclude your main application class itself.
@ WebMvcTest# excludeFilters to exclude configuration classes by their packages.
However, none of these attempts may yield the desired results. Why is that? Let’s break it down.
Why Previous Methods Didn’t Work
Normal Configuration vs. Auto-Configuration:
FooConfiguration and BarConfiguration are standard configuration classes, not auto-configuration classes.
The excludeAutoConfiguration annotation only works on auto-configuration classes, which does not apply here.
Component Scanning and Exclude Filters:
The excludeFilters attribute is part of @ ComponentScan.
It applies only when the configuration classes are recognized via component scanning. Since these configurations are imported explicitly using @ Import, they are not covered by this mechanism.
The Solution: Using Profiles
A simple yet effective way to resolve this problem is by leveraging the @ Profile annotation to conditionally manage configuration imports based on the active profile. Here’s how it works.
Step-by-Step Solution:
Create a New Configuration Class:
Introduce an ExternalModuleConfiguration that includes the necessary configurations but conditionally based on the profile.
[[See Video to Reveal this Text or Code Snippet]]
Modify the Main Application Class:
Import the ExternalModuleConfiguration in your main Spring Boot application.
[[See Video to Reveal this Text or Code Snippet]]
Set Active Profile in Your Test:
In your test class, specify that the active profile is test, ensuring ExternalModuleConfiguration won’t be loaded.
[[See Video to Reveal this Text or Code Snippet]]
Summary
By using the @ Profile annotation, you can control when certain configurations are loaded in your Spring Boot application. This approach helps ensure that during tests, the configurations you do not want are excluded, allowing for a focused and clean testing approach. By following the steps outlined above, you can effectively manage imported configurations in your @ WebMvcTest, leading to more robust and reliable tests.
In Conclusion
Excluding imported configurations from your Spring Boot application during tests might seem daunting at first due to the complexities of the framework. However, with the correct use of profiles, you can simplify your testing setup significantly. Now, you're equipped with the knowledge to streamline your testing process. Happy coding!
コメント