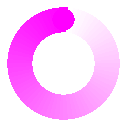
NullSafe Ways to Concatenate Two Streams in Java
Learn how to safely concatenate two streams in Java 8 without encountering null pointer exceptions. Discover efficient methods to handle nullable streams.
---
This video is based on the question stackoverflow.com/q/68912965/ asked by the user 'Jishnu Prathap' ( stackoverflow.com/u/3651739/ ) and on the answer stackoverflow.com/a/68913030/ provided by the user 'Eugene' ( stackoverflow.com/u/1059372/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: What is NullSafe way to concatenate two Streams?
Also, Content (except music) licensed under CC BY-SA meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
NullSafe Ways to Concatenate Two Streams in Java
In Java, especially when working with streams introduced in Java 8, dealing with null values can lead to runtime exceptions that disrupt the smooth flow of your application. One common scenario developers face is trying to concatenate two streams, where one or both streams may be null. This raises the question: What is the best way to concatenate two streams while ensuring that you do not encounter a null pointer exception?
Understanding the Problem
When combining two Stream<Integer> objects, such as guests and guestsTravelWith, a null value in either stream will lead to a NullPointerException. This can be frustrating and often leads developers to add cumbersome if conditions throughout their code. Luckily, there are more elegant and straightforward solutions available that adhere to the principles of null safety.
Exploring NullSafe Solutions
Let’s dive into some practical approaches to concatenating two streams without running into null-related issues.
Solution 1: Using Stream.ofNullable
Java 8 offers a clever method that allows for null-safe operations called Stream.ofNullable(). This method will take an object and return an empty stream if the object is null. Here’s how to use it in your concatenation:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code:
Stream.ofNullable(guestsTravelWith): This will create a stream from guestsTravelWith, or return an empty stream if it’s null.
.orElse(Stream.empty()): This ensures that if the first stream is null, an empty stream will be used in its place.
This logic is applied to both streams being concatenated.
Using this method not only eliminates the risk of errors but also maintains clean and readable code.
Solution 2: Using Ternary Operator
Another straightforward approach is to use a ternary operator to check for null values:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code:
The ternary operator checks if guestsTravelWith or guests is null and assigns an empty stream if they are.
Then, it concatenates the two secure streams.
Best Practices
While the methods above effectively handle concatenating nullable streams, it’s also essential to evaluate your overall stream-returning methods. Consider revising your design if you frequently find yourself returning null streams:
Return Optional Stream or Empty Stream:
Aim to design your methods to return a safe stream, either a proper stream of values or an empty one, instead of null.
Conclusion
By implementing these null-safe approaches to concatenating streams, you can significantly reduce the risk of encountering NullPointerException in your Java applications. Whether you opt for Stream.ofNullable() or a concise ternary operator, maintaining null safety is crucial for robust and stable software development.
Now that you have these methods at your disposal, you can confidently handle streams without worrying about null values ruining your day!
コメント