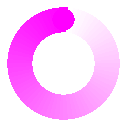
Efficiently Filter a numpy.dstack Without Loops
Learn how to filter a numpy.dstack array using slicing methods instead of loops for better performance and simplicity in Python.
---
This video is based on the question https://stackoverflow.com/q/75057926/ asked by the user 'benedetta' ( https://stackoverflow.com/u/17002230/ ) and on the answer https://stackoverflow.com/a/75057969/ provided by the user 'Guy' ( https://stackoverflow.com/u/5168011/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Filtering a numpy.dstack
Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/l...
The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Filtering a numpy.dstack: A Simple Guide
When working with multidimensional arrays in Python, specifically using the NumPy library, you might find yourself needing to filter out specific elements based on certain conditions. A common scenario is filtering a dstack (depth stack) array based on the values of a specified component. In this post, we will explore how to efficiently filter a numpy.dstack array while ensuring that entire rows of data meet the specified condition.
Understanding the Scenario
Imagine you have created a numpy.dstack using three 1D arrays. Here’s an example of such an array:
[[See Video to Reveal this Text or Code Snippet]]
In the above structure, stack is a 3D array (or depth stack) where each inner array corresponds to a set of elements from a, b, and c. Your goal is to filter out entire rows where the second component (index 2) is less than 1. In other words, if one of the elements in the second position of a row is less than 1, discard that entire row from the final output.
The Common Approach: Using Loops
Many might initially attempt to solve this problem with loops as illustrated below:
[[See Video to Reveal this Text or Code Snippet]]
While this will filter based on conditions, it won’t achieve a structured output containing the required rows. Instead, it only collects the desired elements without regard for their original structure.
The Improved Solution: Slicing
The beauty of NumPy lies in its ability to handle operations on entire arrays without the need for explicit loops. Instead of iterating through each element, slicing offers a much cleaner and efficient solution.
Step-by-Step Filtering Using Slicing:
Identify the Condition: We need to filter based on the condition that the elements in the second position are greater than or equal to 1.
Apply Slicing: You can filter the entire stack array based on the condition directly with the following line of code:
[[See Video to Reveal this Text or Code Snippet]]
This gives us a filtered version of the stack, maintaining the full rows that meet the condition.
Reshaping the Output (if needed)
If your requirement is to reshape this filtered output into a specific 3D format, you can easily do that with additional methods. For instance, to reshape it into a format where each row is encapsulated as a separate layer:
[[See Video to Reveal this Text or Code Snippet]]
Here we have applied the reshape method to get a structured representation that meets your formatting needs.
Conclusion
Using slicing in NumPy not only streamlines your code but also significantly enhances its performance, especially with larger datasets. Whether you are filtering based on conditions or reshaping the results, embracing the strengths of NumPy can save you time and produce cleaner, more efficient results. Consider applying these techniques in your own projects to see how they simplify your data handling. Happy coding!
コメント