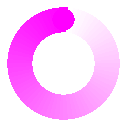
Difference between Java Constructor and Python constructor #python #java #machinelearning
Java and Python both have constructors. Here's a comparison below:
1. Syntax:
Java:
A constructor is a special method with the same name as the class.
class MyClass {
int x;
// Constructor
MyClass(int y) {
x = y;
}
}
Python:
The constructor method is named __init__.
It must have self as its first parameter.
class MyClass:
def __init__(self, y):
self.x = y
2. Overloading:
Java:
Java supports constructor overloading, allowing multiple constructors with different parameter lists.
class MyClass {
int x;
String y;
MyClass(int x) {
this.x = x;
}
MyClass(int x, String y) {
this.x = x;
this.y = y;
}
}
Python:
Python does not support constructor overloading in the same way. Instead, you can use default arguments or conditional logic within the _init_ method.
class MyClass:
def __init__(self, x, y=None):
self.x = x
if y:
self.y = y
3. Inheritance:
Java:
You can call the superclass's constructor explicitly using super().
Python:
To call the superclass's constructor, use super().__init__().
4. Object Creation:
Java:
MyClass obj = new MyClass(10);
Python:
obj = MyClass(10)
5. Constructor Chaining:
Java
class MyClass {
int x;
String y;
MyClass(int x) {
this(x, "Default");
}
MyClass(int x, String y) {
this.x = x;
this.y = y;
}
}
Python:
class MyClass:
def __init__(self, x, y="Default"):
self.x = x
self.y = y
.
.
.
.
.
.
.
.
.
#python #java #codinglife #googlecolab #programmerlife #codingpics #coders #googlebug #tcs #programmingisfun #constructor #coding #coderpower #love #interview #coder #infosys #viralreel #google #dsa #codergirl #programming #datastructure #cpp #coderslife #computerscience #programmingjokes #machinelearning #ashishgadpayle #ashishgadpaylecodingjavasql
コメント