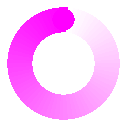
Binary search tree data structures in python 5
Download 1M+ code from codegive.com/2bfdfd6
binary search trees in python 5: a comprehensive tutorial
binary search trees (bsts) are fundamental data structures in computer science, providing efficient ways to store and retrieve data in a sorted manner. this tutorial will delve into the concepts behind bsts, their implementation in python 5, common operations, and potential use cases.
*1. understanding the basics*
a binary search tree is a tree-based data structure that adheres to the following properties:
*binary tree:* each node in the tree has at most two children, referred to as the left child and the right child.
*search property:* for any node in the tree:
all nodes in its left subtree have values less than the node's value.
all nodes in its right subtree have values greater than the node's value.
this property allows for efficient searching, insertion, and deletion operations.
*visual representation:*
in this example, the root node is 8. notice how all nodes to the left of 8 (3, 1, 6, 4, 7) are less than 8, and all nodes to the right (10, 14, 13) are greater than 8.
*2. implementing a binary search tree in python 5*
we'll start by defining a `node` class and a `binarysearchtree` class.
*explanation:*
*`node` class:* represents a single node in the tree. it stores the `data` and references to its `left` and `right` children.
*`binarysearchtree` class:* represents the entire bst.
`__init__`: initializes an empty tree by setting `root` to `none`.
`insert(data)`: inserts a new node with the given data. it calls the `_insert_recursive` method to handle the actual insertion process. if the tree is empty, it creates a new `node` and sets it as the root. handles duplicates by printing a warning (can be customized to suit your needs).
`_insert_recursive(data, current_node)`: recursively traverses the tree to find the correct position to insert the new node based on the bst property.
`search(data)`: searche ...
#BinarySearchTree #PythonDataStructures #CodingTutorials
binary search tree
BST
Python data structures
tree traversal
insertion algorithm
deletion algorithm
node balancing
AVL tree
red-black tree
recursive functions
tree height
depth-first search
breadth-first search
data organization
dynamic data structures
コメント