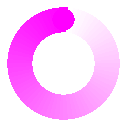
@ExceptionHandler REST Error Handling With Spring Boot : 2018
In this video we are going to learn about the exception handling mechanisms in Spring Boot
Before jumping on to explaining this lets have a clarification about the differences between an error and an exception.
An error is something wrong that has happened in the program and the program cannot progress further, it is a blocker.
Whereas an exception is something that is not mandatorily needs to be addressed and program can progress even if the issue persists.
So what we do is we handle that exception programmatically like a simple try catch in Java.
Now, from the Spring Boot perspective assume your code has hit the exception. What will happen?
If exception occurs and your code doesn't take care of that, you actually need not worry because Spring Boot as a framework provides
a default exception handling mechanism.
Please see the code snippet
{
"timestamp": "2018-08-18T04:28:20.277+0000",
"status": 500,
"error": "Internal Server Error",
"message": "could not execute statement; nested exception is org.hibernate.exception.ConstraintViolationException: could not execute statement",
"path": "/users"
}
To display this kind of document their is no need of creating a JSON document or a Java class.
Now the question is How to handle the exception of REST web services app built with Spring Boot?
This is very simple and easy, its just that we need to follow a procedure.
1. Create a new class annotated with @ControllerAdvice
2. Add a method that is annotated with @ExceptionHandler specifying which Exception class this method needs to handle.
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.context.request.WebRequest;
import org.springframework.web.servlet.mvc.method.annotation.ResponseEntityExceptionHandler;
@ControllerAdvice
public class EntityExceptionHandler {
@ExceptionHandler(value = { Exception.class })
public ResponseEntity[Object] handleAllException(Exception ex, WebRequest request) {
return new ResponseEntity[](
ex.getMessage(), new HttpHeaders(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
This code can handle any type of exceptions ok
Now one more situation. How to handle a custom type of exception happened at Runtime
Take a look at this code now
public class MyException extends RuntimeException{
private static final long serialVersionUID = 5212338953864776681L;
public MyException (String message)
{
super(message);
}
}
So now we have created our own exception class lets try to catch it.
@ControllerAdvice
public class MyHandler extends ResponseEntityExceptionHandler {
@ExceptionHandler(MyException.class)
public final ResponseEntity[ErrorMessage] specificException(Exception ex, WebRequest request) {
ErrorMessage errorMessage = new ErrorMessage(new Date(), ex.getMessage(),
request.getDescription(false));
return new ResponseEntity[angularbrackets](errorMessage, new HttpHeaders(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
So if you carefully observe, we are passing the name of the class file with @ExceptionHandler annotation
Using, this we can handle a custom type exception.
To recap first I explained what is the difference between an error and an exception, then we tried to handle any kind of
exception using Spring Boot and finally we explained you how to handle your own custom exception by passing the name of the class name.
By using these, we can handle almost any type of exception you want.
Thank you for watching this video
Please read [ ] for angular brackets.
コメント