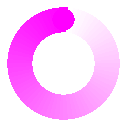
How to Sort a List of Strings by Date Substrings in Python
Learn how to effectively sort a list of strings that contain dates in the format yyyymmdd using Python. This guide will walk you through the process step-by-step.
---
This video is based on the question stackoverflow.com/q/70207490/ asked by the user 'Tom Hardy' ( stackoverflow.com/u/16970229/ ) and on the answer stackoverflow.com/a/70207530/ provided by the user 'rchome' ( stackoverflow.com/u/12005414/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions.
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How do I sort a list of strings by the substring date?
Also, Content (except music) licensed under CC BY-SA meta.stackexchange.com/help/licensing
The original Question post is licensed under the 'CC BY-SA 4.0' ( creativecommons.org/licenses/by-sa/4.0/ ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( creativecommons.org/licenses/by-sa/4.0/ ) license.
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Sort a List of Strings by Date Substrings in Python
Sorting data is a common task in programming, and sometimes, we encounter specific challenges that require a tailored approach. One such challenge arises when we want to sort a list of strings based on a date embedded in each string. If you've ever received a list of links with dates formatted as yyyymmdd, you might be wondering how to sort them effectively. In this guide, we will dive into a straightforward solution to this problem.
Understanding the Problem
You have a list of links, and within each link, there exists a date string formatted as yyyymmdd. Your goal is to sort these links by the date portion, which is typically located at a specific index within the string. Let's imagine the following links as an example:
example.com/file_20230101.pdf
example.com/file_20221231.pdf
example.com/file_20231111.pdf
To effectively sort this list by date, rather than converting these date strings to a datetime object, we can leverage Python's inherent string sorting capabilities.
Proposed Solution
Step 1: Identify the Date Substring
First, it’s key to know where the date is located within the strings. In the example above, the date substring can be accessed using a specific index range. Since we are dealing with the format yyyymmdd, let’s assume the date begins at index 48 and ends at index 56 for each link string.
Step 2: Use the Sorted Function
The most efficient way to sort these strings by the date substring is by using Python's built-in sorted() function. This function allows us to specify a custom sorting key using a lambda function. Here’s how you can do it:
[[See Video to Reveal this Text or Code Snippet]]
Detailed Explanation
Lambda Function: A lambda function is an anonymous function defined with the lambda keyword. In our case, lambda l: l[48:56] extracts the date substring from each link.
Key Parameter: The key parameter of the sorted() function allows us to pass the lambda function, which will be executed for each element in the list. The result of this function will determine the order of the elements.
No Need for Conversion: Since the date is formatted in a way that the string comparison aligns with chronological order, we do not need to convert it to a datetime object.
Example Usage
Now, let’s see how this can be implemented in practice. Here’s a full example that includes the function and some sample links:
[[See Video to Reveal this Text or Code Snippet]]
Result
When you run the above code, you will get the links sorted in chronological order based on their date substring:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Sorting a list of strings by a date substring, such as yyyymmdd, can be accomplished with elegant simplicity in Python. By using the sorted() function along with a lambda expression, you can effectively sort your links without the need to convert the strings to dates. This approach not only saves time but also keeps your code clean and readable.
Now that you have a clear understanding of how to tackle this issue, you can confidently sort your date-embedded strings in Python. Happy coding!
コメント